Bash is a command processor that typically runs in a text window, allowing the user to type commands that cause actions. Bash reads commands through the file, known as a script. Bash scripts are incredibly versatile and can be used to automate a wide range of tasks. One particularly useful feature is the ability to create a multiple choice menu, which can make your scripts more interactive and user-friendly.
In this blog post, we’ll explore how to create a multiple choice menu in Bash scripts, which can be a great addition to any developer’s toolkit.
Table of Content
How to Create a Multiple Choice Menu in Bash Scripts
Before diving into the creation of a multiple choice menu, it’s important to understand some basic concepts. To create a multiple choice menu in Bash, we primarily use two constructs. select is the core command for creating the menu itself. case handle the user’s choice.
Create a Bash Script
Create a new file with a .sh extension (e.g., menu.sh). Open it in a text editor such as nano:

Setting Up the Input Prompt
The first step in creating a multiple choice menu is to set up the input prompt. This is done using the PS3 variable, which defines the prompt string displayed to the user when the select command is used. For example:
PS3='Please select an option: '
Creating Your List of Options
Next, you’ll need to create an array of options for the user to choose from. This is done by defining an array variable with the list of choices. Let’s create an array to carry different menu options as below:
#!/bin/bash
options=("Option 1" "Option 2" "Option 3" "Quit")
Crafting the Menu with select
Use the select loop to display the menu and capture the user’s choice. The select construct is used to generate the menu. It takes the list of options and generates a menu from them. Here’s how you can use select to create your menu:
select opt in "${options[@]}"
do
# Your code goes here
done
Implementing case Statements
To handle the user’s selection, case statements are used. These allow you to execute different pieces of code based on the user’s input. Here’s an example of how case statements can be used within the select construct:
select opt in "${options[@]}"
do
case $opt in
"Option 1")
echo "You chose option 1"
;;
"Option 2")
echo "You chose option 2"
;;
"Option 3")
echo "You chose option 3"
;;
"Quit")
break
;;
*)
echo "Invalid option $REPLY"
;;
esac
done
Code Description:
- First, evaluates the user’s choice ($opt) and performs actions based on the selected option.
- Then, Option 1, 2, or 3 prints a confirmation message.
- After that, Quit exits the program.
- Finally, the invalid option displays an error message with the selected option.
Complete Code
Now that we’ve covered the individual components, let’s put everything together into a complete script:
#!/bin/bash
# Set up the input prompt
PS3='Please select an option: '
# Create the list of options
options=("Option 1" "Option 2" "Option 3" "Quit")
# Generate the menu and handle user input
select opt in "${options[@]}"
do
case $opt in
"Option 1")
echo "You chose option 1"
;;
"Option 2")
echo "You chose option 2"
;;
"Option 3")
echo "You chose option 3"
;;
"Quit")
break
;;
*)
echo "Invalid option $REPLY"
;;
esac
done
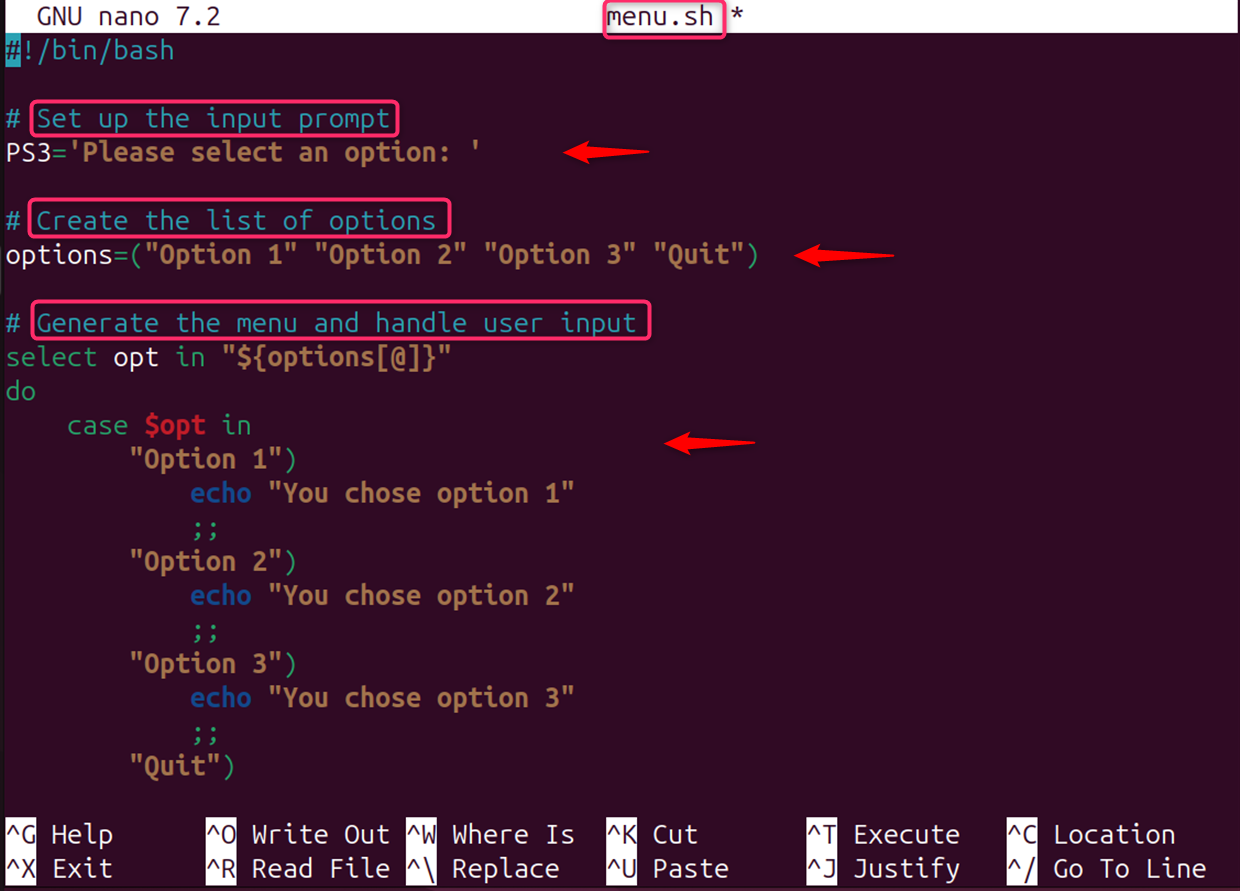
Code Description
- First, the PS3 sets the prompt for the menu.
- Then, select opt in “${options[@]}” and create a menu with the options from the array.
- After that, case $opt in checks the user’s choice and executes corresponding code.
- In addition, break exits the loop when “Quit” is selected.
- Finally, *) handles invalid choices.
Make the Script Executable
The code chmod +x menu.sh is a command in the Bash shell used to change the permissions of a file named menu.sh. +x is an option for the chmod command. Here, the + symbol signifies adding permissions, and x represents the execute permission.
chmod +x menu.sh

So, combining these parts, chmod +x menu.sh instructs the system to add the execute permission to the file menu.sh.
Run the Script
This script provides a basic structure for creating a user-friendly, interactive menu in your Bash scripts. The code in the script ./menu.sh creates a text-based multiple choice menu for the user:
./menu.sh
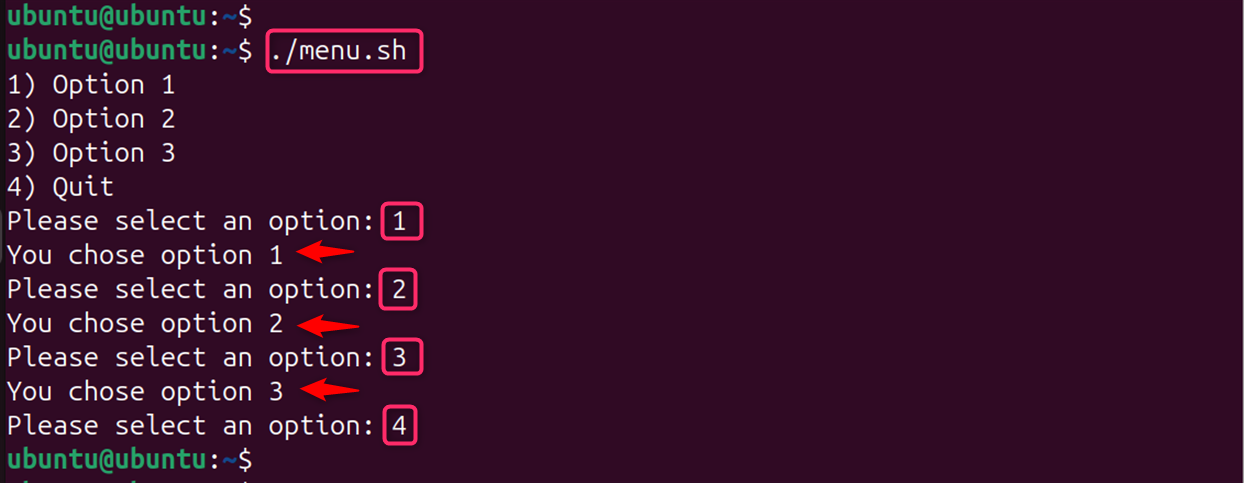
This script displays a menu with three options and a quit option. When the user selects an option, the corresponding message is displayed. When the user selects “Quit“, the break statement exits the select loop, effectively terminating the script.
By following these steps and incorporating the provided code, you can effectively create multiple choice menus in your Bash scripts.
Conclusion
Creating a multiple choice menu in Bash scripts is a straightforward process that can greatly enhance the interactivity and user-friendliness of your scripts. By following the steps outlined in this post, you can easily implement such menus in your own Bash scripts. For more detailed examples and advanced usage, you can refer to online resources and tutorials that provide in-depth explanations and additional context.
Leave feedback about this